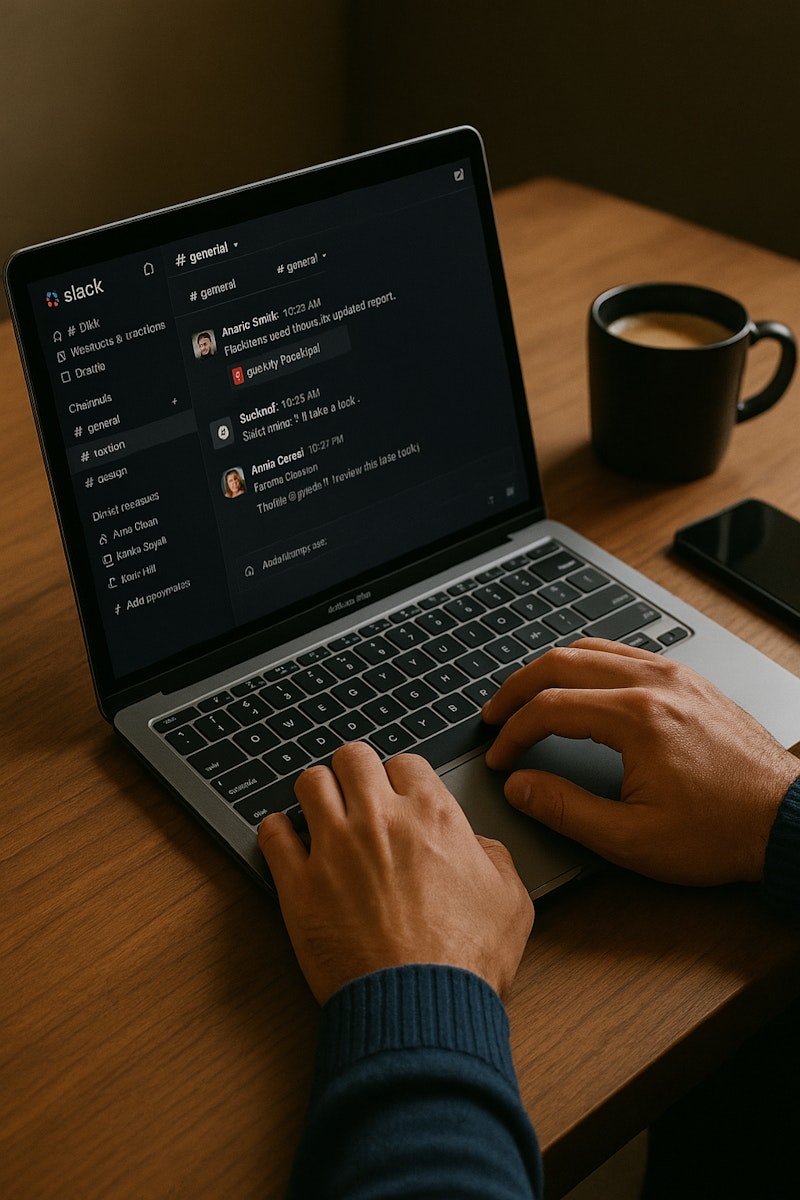
5 May, 2025
Mastering Laravel Email: Configuration and Command-Line Testing
Luigi Laezza
5 minutes
Web Development
Email remains a cornerstone of modern web applications, facilitating user communication, notifications, and crucial system alerts. Laravel, with its elegant syntax and powerful features, simplifies the process of setting up and sending emails. This comprehensive guide will walk you through the intricacies of configuring Laravel's email capabilities and demonstrate how to send a quick test email directly from your command line.
Understanding Laravel's Mail System
Laravel leverages the robust SwiftMailer library (now Symfony Mailer) under the hood, providing a consistent and flexible API for sending emails. It supports various mail drivers, allowing you to choose the most suitable option for your application's needs, ranging from local development to production environments.
Key Components:
- Mail Configuration: The foundation of Laravel's email system lies in its configuration files, primarily config/mail.php. This file houses settings for your default mailer, mail drivers, and global "from" addresses.
- Mail Drivers: Laravel offers several built-in mail drivers:
- smtp: Sends emails via a Simple Mail Transfer Protocol (SMTP) server. This is a common choice for production environments.
- mail: Utilizes PHP's built-in mail() function. Suitable for basic local development but often lacks features and reliability for production.
- sendmail: Sends emails by invoking the sendmail binary on your server. Another option for server-based email delivery.
- log: Writes all email messages to your Laravel log files. Ideal for debugging and local development where you don't want to send actual emails.
- array: Stores sent emails in an array, primarily used for testing purposes.
- failover: Allows you to define multiple mailers and automatically switch to the next one if the current one fails.
- ses: Specifically designed for Amazon Simple Email Service (SES).
- mailgun: Integrates with the Mailgun email service.
- postmark: Supports the Postmark transactional email service.
- Mailables: Laravel introduces the concept of "Mailables," which are classes that represent your email messages. They encapsulate the email's subject, recipient(s), content (both text and HTML), attachments, and any custom headers. This object-oriented approach promotes cleaner and more maintainable email logic.
- Mail Facade: Laravel provides the Mail facade, a simple and expressive way to interact with the mail sending functionality without needing to instantiate mailer classes directly.
Step-by-Step Guide to Setting Up Laravel Email
- Configure Your .env File: The primary configuration for your mail setup resides in your .env file. Open this file in your project root and look for the MAIL_* variables. Adjust these based on your chosen mail driver.
- For SMTP (most common for production):
- Code snippet
MAIL_MAILER=smtp MAIL_HOST=your_smtp_host.com MAIL_PORT=your_smtp_port MAIL_USERNAME=your_smtp_username MAIL_PASSWORD=your_smtp_password MAIL_ENCRYPTION=tls # or ssl [email protected] MAIL_FROM_NAME="${APP_NAME}"
- Replace the placeholder values with your SMTP server details provided by your email hosting provider. Ensure the port and encryption match their requirements. The MAIL_FROM_ADDRESS and MAIL_FROM_NAME will be the default sender for your application's emails.
- For Mailgun:
- Code snippet
MAIL_MAILER=mailgun MAILGUN_DOMAIN=your_mailgun_domain MAILGUN_SECRET=your_mailgun_secret MAILGUN_ENDPOINT=api.mailgun.net # or a regional endpoint [email protected] MAIL_FROM_NAME="${APP_NAME}"
- Obtain your Mailgun domain and secret from your Mailgun dashboard.
- For Amazon SES:
- Code snippet
MAIL_MAILER=ses AWS_ACCESS_KEY_ID=your_aws_access_key_id AWS_SECRET_ACCESS_KEY=your_aws_secret_access_key AWS_DEFAULT_REGION=your_aws_region # e.g., us-east-1 [email protected] MAIL_FROM_NAME="${APP_NAME}"
- Ensure you have your AWS credentials and the correct region configured.
- For mail (primarily for local development):
- Code snippet
MAIL_MAILER=mail [email protected] MAIL_FROM_NAME="${APP_NAME}"
- No further configuration is typically needed for the mail driver, assuming your server has a properly configured mail transfer agent.
- For log (local development and debugging):
- Code snippet
MAIL_MAILER=log [email protected] MAIL_FROM_NAME="${APP_NAME}"
- All emails will be written to your Laravel log files (storage/logs/laravel.log).
- Clear Configuration Cache: After modifying your .env file, it's crucial to clear Laravel's configuration cache to ensure the new settings are loaded:
- Bash
php artisan config:clear php artisan config:cache
- (Optional) Configure config/mail.php: While the .env file handles most common configurations, you can further customize your email settings in the config/mail.php file. Here, you can:
- Change the default mailer.
- Define specific configurations for different mailers within the mailers array.
- Set a global "from" address that can be overridden in individual Mailables.
- Configure mailer-specific options (e.g., transport options for SMTP).
Sending a Test Email from the Command Line with Laravel Tinker
As you mentioned, Laravel Tinker provides an excellent way to quickly test your mail configuration without interacting with your application's front-end or triggering specific events.
- Access Laravel Tinker: Open your terminal or SSH into your server, navigate to the root directory of your Laravel project, and run the Tinker command:
- Bash
php artisan tinker
- This will open an interactive PHP shell with access to your Laravel application's environment and services.
- Utilize the Mail Facade: Within the Tinker session, you can use the Mail facade to send a raw text email for a quick test. The Mail::raw() method allows you to specify the email body and a closure that defines the recipient and subject.
- PHP
Mail::raw('This is a test email sent from Laravel Tinker!', function ($message) { $message->to('[email protected]')->subject('Laravel Test Email'); });
- Explanation:
- Mail::raw('This is a test email sent from Laravel Tinker!', ...): This calls the raw() method of the Mail facade. The first argument is the plain text body of the email.
- function ($message) { ... }: This is a closure that receives a $message object, which is an instance of Illuminate\Mail\Message. This object provides methods for configuring the email.
- $message->to('[email protected]'): This sets the recipient's email address. Remember to replace '[email protected]' with your actual email address.
- ->subject('Laravel Test Email'): This sets the subject line of the email.
- Customize Sender (Optional): You can further customize the sender's email address and name using the $message->from() method:
- PHP
Mail::raw('This is a test email with a custom sender.', function ($message) { $message->from('[email protected]', 'Test Sender Name') ->to('[email protected]') ->subject('Test Email with Custom Sender'); });
- Send the Email: After entering the Mail::raw() command, press Enter. Laravel will attempt to send the email using the configured mail driver.
- Check for Success or Errors:
- If the email is sent successfully (depending on your driver), Tinker might output null or true.
- If there are any configuration issues or errors with your mail setup, Tinker will likely display an exception or error message, providing valuable clues for troubleshooting. Check your Laravel logs (storage/logs/laravel.log) for more detailed error information.
- Exit Tinker: Once you've finished testing, you can exit the Tinker session by typing exit or pressing Ctrl + D.
Beyond Mail::raw(): Using Mailables
For more complex emails with dynamic data, HTML content, attachments, and a more structured approach, Laravel's Mailables are highly recommended.
- Generate a Mailable: Use the Artisan command to create a new Mailable class:
- Bash
php artisan make:mail TestMail
- This will create a file in app/Mail/TestMail.php.
- Define the Mailable: Open the generated TestMail.php file and customize its properties and the build() method.
- PHP
<?php namespace App\Mail; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Mail\Mailable; use Illuminate\Mail\Mailables\Content; use Illuminate\Mail\Mailables\Envelope; use Illuminate\Queue\SerializesModels; class TestMail extends Mailable { use Queueable, SerializesModels; public $data; /** * Create a new message instance. * * @return void */ public function __construct(array $data) { $this->data = $data; } /** * Get the message envelope. */ public function envelope(): Envelope { return new Envelope( subject: 'Test Email from Mailable', ); } /** * Get the message content definition. */ public function content(): Content { return new Content( markdown: 'emails.test', // Assuming you have a Blade template at resources/views/emails/test.blade.php with: [ 'name' => $this->data['name'], 'message' => $this->data['body'], ], ); } /** * Get the attachments for the message. * * @return array */ public function attachments(): array { return []; } }
- Create the Email View (if using Markdown or Blade): If you specified a Markdown or Blade template in the content() method, create the corresponding view file (e.g., resources/views/emails/test.blade.php):
- Blade
<x-mail::message> # Hello {{ $name }}! {{ $message }} Thanks,<br> {{ config('app.name') }} </x-mail::message>
- Send the Mailable via Tinker: In your Tinker session, you can now instantiate and send your Mailable:
- PHP
$data = ['name' => 'John Doe', 'body' => 'This is a test email sent using a Mailable.']; Mail::to('[email protected]')->send(new App\Mail\TestMail($data));
- This demonstrates how to pass data to your Mailable, which can then be used in your email views.
Troubleshooting Common Email Issues
- Incorrect SMTP Credentials: Double-check your SMTP host, port, username, password, and encryption settings in your .env file.
- Firewall Blocking Ports: Ensure that your server's firewall allows outbound connections on the SMTP port (typically 465 for SSL or 587 for TLS).
- Email Marked as Spam: Check your spam folder. Ensure your domain has proper SPF and DKIM records configured to improve email deliverability.
- Server Not Allowed to Send Email: Some hosting providers restrict outbound email traffic. Contact your provider to verify if any restrictions are in place.
- Configuration Cache Issues: Always clear your configuration cache after modifying .env or config/mail.php.
- Log Files: Examine your Laravel log files (storage/logs/laravel.log) for detailed error messages.